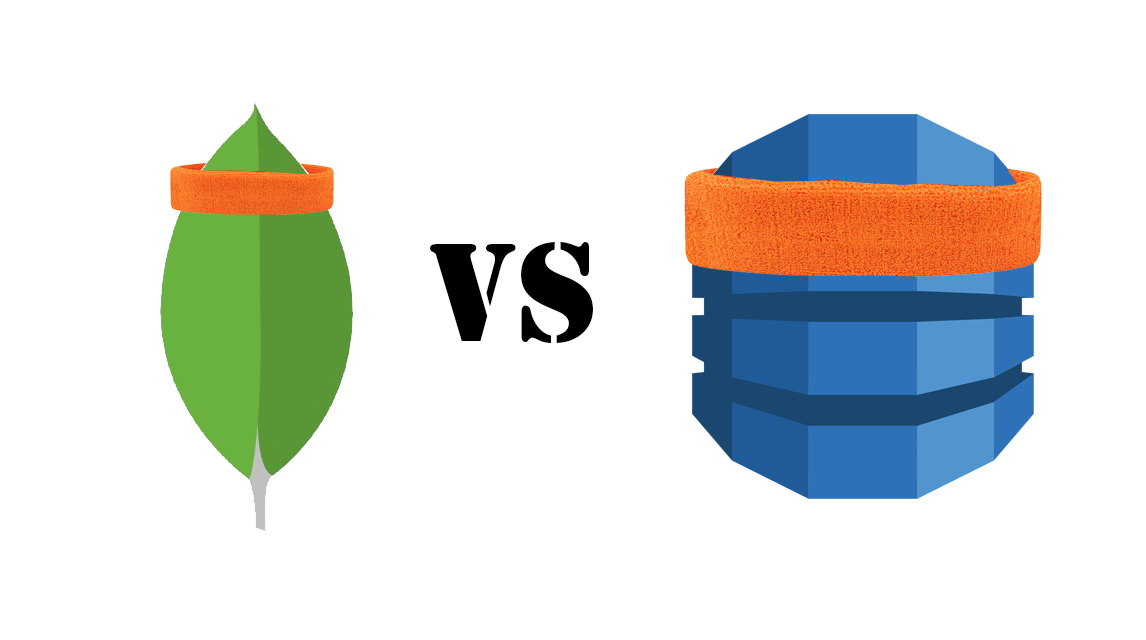
Here at Optimal, we are currently working on some microservice management software. Part of my work on this project has been implementing some database classes for message brokers to interact with. So far I’ve implemented classes for DynamoDB and MongoDB: two NoSQL databases with their own strengths and weaknesses.
NoSQL is pretty much what it sounds like: databases that don’t use SQL. NoSQL databases are not relational, that is, there are no connections between tables in a database.
In this blog, we’ll look at factors of cost, security and ease-of-use.
Let’s get ready to rumble!
Setting Up
DynamoDB
Setting up Dynamo was a breeze. Because it’s an Amazon Web Service, it’s just a matter of going to the AWS console and tottering through a wizard to create your database.
MongoDB
Welcome to hell, kids. Setting up a Mongo database is a sea of terminal text and unhelpful authentication errors. I had a tonne of problems getting started with Mongo, to the extent that it took me over a day to get it going. Sometimes fixing a problem was as small as correcting a typo, but at other times is was a matter of uninstalling and starting from scratch.
MongoDB has some instructions for getting set up which are helpful if you’re fluent in the Ubuntu terminal:
However, there are a few things missing from these guides that required some intense googling. Tim and I have put together a guide for installing MongoDB, which may turn into a blog. Watch this space.
However, you can pay for MongoDB Atlas which is cloud hosted and, according to their website, “The easiest way to run MongoDB”. I haven’t tried this out, so perhaps it puts Mongo on a similar level to Dynamo here. You could easily argue that we’re comparing apples and oranges in this case.
Programming
Both of these database tools have libraries for use in C#. I worked with each of them.
DynamoDB
Have you ever wanted to throw your PC out of the window of a multi-storey building? Try using the Dynamo library and you will.
I found programming with the DynamoDB library to be unintuitive and frustrating. Nothing works the way you expect it to. If you are planning on programming synchronously, you’re in for a ride. All of Dynamo’s basic methods like creating tables and adding data to them are asynchronous.
Here’s the code for creating a table with MongoDB:
[code language=”csharp”]this.collection = db.GetCollection<BsonDocument>(tableName);[/code]vs creating a table with DynamoDB:
[code language=”csharp”]public void CreateTable(string tableName) {
try {
Task<CreateTableResponse> response = client.CreateTableAsync(new CreateTableRequest {
TableName = tableName,
AttributeDefinitions = new List<AttributeDefinition>() {
new AttributeDefinition {
AttributeName = "Id",
AttributeType = "S"
}
},
KeySchema = new List<KeySchemaElement>() {
new KeySchemaElement {
AttributeName = "Id",
KeyType = "HASH"
}
},
ProvisionedThroughput = new ProvisionedThroughput {
ReadCapacityUnits = readCapacity,
WriteCapacityUnits = writeCapacity
}
});
WaitTillTableCreated(response.Result, tableName);
} catch(AggregateException e) {
OLog.Info("Cannot create table as it already exists.");
}
}
private void WaitTillTableCreated(CreateTableResponse response, string tableName) {
TableDescription tableDescription = response.TableDescription;
string status = tableDescription.TableStatus;
// Wait until table is created.
while(status != "ACTIVE") {
System.Threading.Thread.Sleep(1000); // Wait 1 second.
try {
Task<DescribeTableResponse> res = client.DescribeTableAsync(new DescribeTableRequest {
TableName = tableName
});
status = res.Result.Table.TableStatus;
}
// Try-catch to handle potential eventual-consistency issue.
catch(ResourceNotFoundException) {}
}
}
[/code]Have you punched a hole in your monitor yet?
To make things worse, there’s a heap of outdated documentation floating around, which makes troubleshooting a minefield. You think you’ve found the method to save you, but try to use it and it comes up deprecated.
Running queries can be a pain too. An ordinary query can only take a primary key as a parameter. As in, you can only use a query to get data about an item if you know what the item is.
“But what if I want to search for all items with a certain property?” I hear you cry.
Well, you can use a scan, but it’s not very smart. It iterates over each row in the table and checks for matches. Pretty slow stuff.
Alternatively, you can use a global secondary index, but that’s a bit of a learning curve in itself. Amazon does provide some good tutorials, however.
MongoDB
The Mongo library was a comparative pleasure to work with. All basic methods exist in both synchronous and asynchronous form. All the methods that should exist, do exist. Querying is as simple is making a list of rules to filter by. It was so intuitive and usable that all but one of my unit tests passed on the first go.
Cost
Both Dynamo and Mongo are freemium, meaning that the services are free up until a certain limit (such as storage space or read/write capacity) or with limited features.
For the paid versions of these services, Dynamo has increasing cost for higher read/write capacity, while Mongo does not. However, Mongo is more expensive to upscale in terms of storage.
Get more info on pricing for DynamoDB and MongoDB.
Security
MongoDB
Security for MongoDB has been in the news a lot lately as it has been at the centre of some ugly database breaches. This is not the fault of MongoDB itself so much as it is MongoDB being used incorrectly.
MongoDB installs with authentication off by default. This is a very bad idea for most users of MongoDB as any data can be changed by anyone at any time. The easiest way to remedy this is to enable authentication via username and password. This can be quick and easy to set up and get going if you know what you’re doing, and only affects the initial connection to the database – none of your other code needs changing. Once this is set, MongoDB has no issues being a nice and secure home for all your data.
Here is a good checklist to make sure you are working securely with MongoDB:
https://docs.mongodb.com/manual/administration/security-checklist/
DynamoDB
DynamoDB’s security is mostly provided by the normal AWS security measures. Access to DynamoDB is granted with IAM through access/secret key pair or roles from the machine the code is running on. Both of these systems are secure and allow easy access to your data!
(Big thanks to Tim for writing this section for me as I had to return to university before I was able to finish it!)
Verdict
And thus the fight comes to an end. And the winner is… subjective?
My personal preference is for MongoDB. It gave me far fewer headaches in the long-run. The ease of using its C# library trumps the difficulty of setting up the free version for me.
However, it’s clear that DynamoDB has its benefits too. If security and plentiful storage are your organisation’s biggest concerns, and then this is the one to go for. Make sure your developers are well-versed in asynchronous programming, however.
It would be nice if the results were as clear as those of a wrestling match, but unfortunately, this is real life, and everything is tinted with shades of grey.
Any choice of software is a matter of what’s the most important to you. Get ready to rumble with your priorities.
Sarah – The tea-drinking programmer