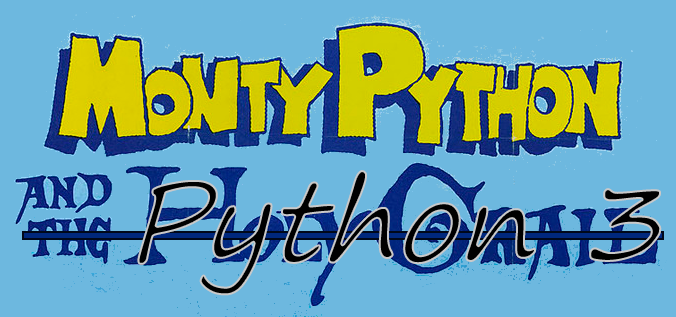
The impending doom of python2 will shortly be upon us (for a dramatic countdown check out the Python clock), so it is time to update our python scripts to Python 3 (3.6 hopefully). Here are a few of the things that made this easier for me over here at OptimalBI. Let’s get into it!
Things to read before we start
First stop is to read up on what things have changed from Python 2 to Python 3.
All the major changes between Python versions are outlined on their website, including between version 2 and 3. It’s long and comprehensive but ensures that you know what you are doing in each version of python.
They also built a cheat sheet for writing code that supports both Python 2 and 3. Handy!
If you still want more and you really love reading there is also a whole BOOK on Python 3. Good times.
Reading one or two of these should set you up to port scripts to the latest version of everyone’s favourite language.
Here’s the ‘too long, didn’t read’ version –
Lots of this is copy pasted from the links above, but here are the big changes you really need to know if you are switching from Python 2 to 3 –
‘print’ is no longer: print "Hello World" it is now print("Hello World"). Simple, and make sense! This shouldn’t affect any major scripts anyway as they should be using Python’s logging framework (they are, right?).
‘dict’s methods now return “views” rather than lists. Think useful methods like dict.keys() and dict.items(). You can cast to a list with dict_list = list(my_dict.items()) or use a method like sorted_dict_list = sorted(my_dict)
You can only compare things that make sense. Ordering comparison now doesn’t work for things that don’t really make sense to compare, so things like 1 < '' , 0 > None or len <= len won’t work.
“Python 3.0 uses the concepts of text and (binary) data instead of Unicode strings and 8-bit strings. All text is Unicode; however encoded Unicode is represented as binary data. The type used to hold text is str, the type used to hold data is bytes.”
Catching exceptions. You must now useexcept SomeException as variable
instead of except SomeException, variable. Moreover, the variable is explicitly deleted when the except block is left.
Anything else I should know?
You don’t necessarily need to do all the changes manually, there is a conversion tool. It’s not 100% and may make some errors, but in certain situations, it’s worth trying out.
If you install it and run 2to3 myscript.py it will print out a nice little diff between the two versions. You can get it to write the changes by adding a -w flag, or make the changes yourself if there are other changes you’d like to make as well.
Unit tests with 80% or greater coverage are also great. If you have unit tests with good coverage you will be able to run them at every stage of upgrading your applications, and quickly catch any bugs before they grow into large annoying bugs.
Oh no, one of my third-party libraries does not support Python 3!
Most Python libraries in pip now support Python 3 and 2 (if not just 3) but there are a few around that only support 2. This is mostly because support for these libraries has been dropped, or the person supporting them has disappeared.
You have a few choices if you run into one of these libraries –
- Contact the developer of the libraries and see if they can update them.
- Find a different library that does the same task (if you have an issue with an old library other people will too!)
- Update them yourself (and open-source it for everyone else). 2to3 really helps here.
- Rewrite the library (or the bits you need) in Python 3.
Of course, these are only options if the library is open source. If it’s a closed source library then you will have to write it from scratch.
That’s it folks, you’re set to start converting from Python 2 to 3. It’s not that hard once you get started.
Happy coding,
Tim Gray
Coffee to Code.