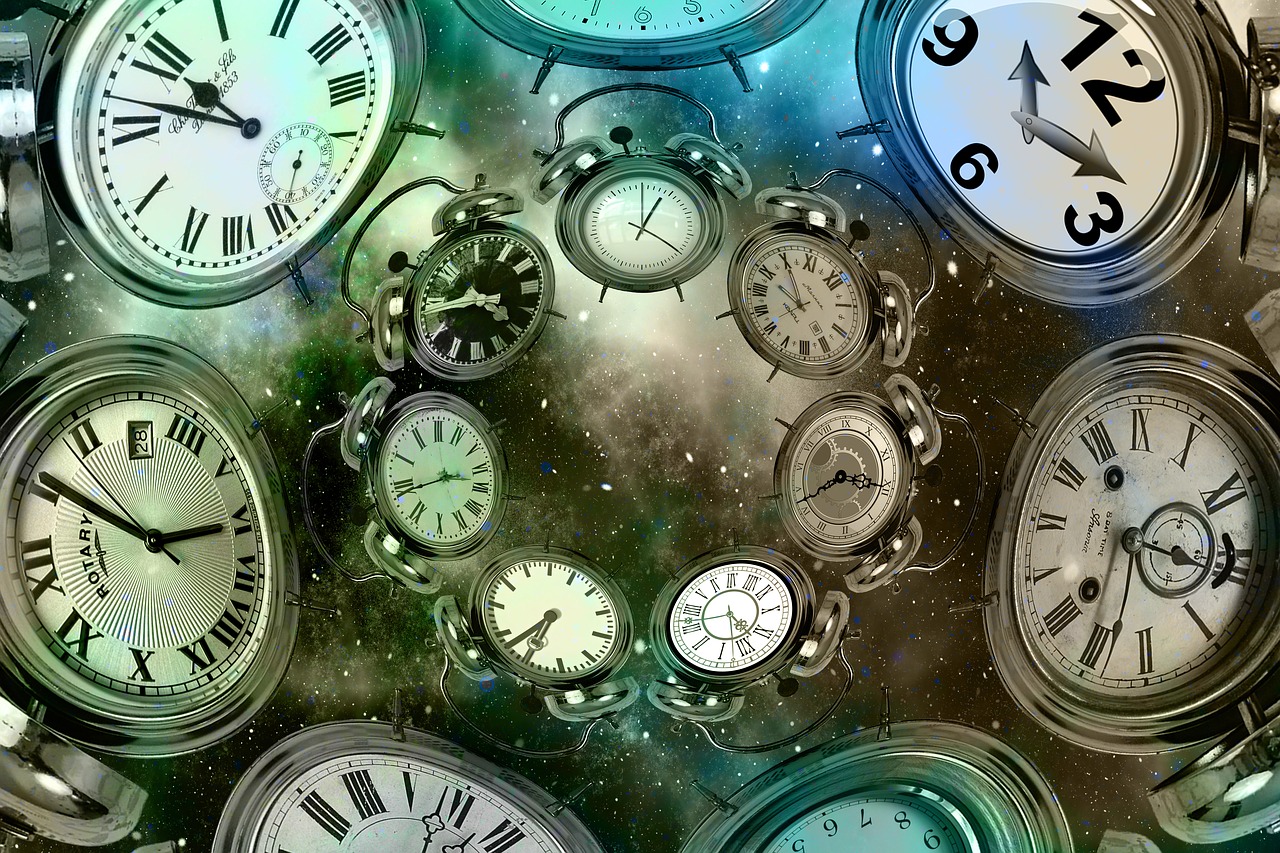
Dealing with times can be quite annoying in programming, but not as annoying as dealing with timezones. Throw in a sprinkling of Daylight Savings Time and you have a good recipe for headaches.
This headache can be very relevant if you are a cloud programmer. Cloud applications often don’t operate in the same Time Zone as their users, so timezone translation is often required to do tasks on appropriate times for your users.
The best medicine for these headaches (as many of you know already) is Moment.js
Note: I will mostly be talking about Node.JS related code in this blog, but Moment.js works exactly the same in browsers and other javascript engines.
Getting started with Moment.js and Moment Timezone
To get started we need to include the moment package into our node project. Simply run:
[code]npm install moment npm
install moment-timezone
[/code]Next we need to add them to our code files, in JavaScript this is as simple as:
[code language=”javascript”]let moment = require("moment");
// OR
let moment = require("moment-timezone");
[/code]If we are using TypeScript (and we should be) then you should also install the “@types/moment” or “@types/moment-timezone” packages to get proper typing information.
We can then include moment in our TypeScript scripts with:
[code language=”javascript”] import * as moment from "moment";
// OR
import * as moment from "moment-timezone";
[/code]Now we are ready to do lots of cool things with date.
[code language=”javascript”]let now = moment(); // This returns the Date/Time of right now.
let normalJavaScriptDate = now.toDate(); // Changes the moment object to a JavaScript Date object.
let dateFormatted = now.format(); // "2014-09-08T08:02:17-05:00" (ISO 8601)
let dateFormattedNice = now.format("dddd, MMMM Do YYYY, h:mm:ss a"); // "Sunday, February 14th 2010, 3:25:50 pm"
moment("20111031", "YYYYMMDD").fromNow(); // 6 years ago
moment().startOf(‘hour’).fromNow(); // 24 minutes ago
[/code]As you can see, doing operations on dates just got a lot simpler.
Timezones
Moment Timezone is the place to go for help with dealing with times across different timezones. Its very easy to use:
[code language=”javascript”]let nowNz = momentTz.tz(date,"Pacific/Auckland"); // Tell moment that this date is a NZ date.
let losAngeles = nowNz.clone().tz("America/Los_Angeles"); // Convert that NZ date to a LA date.
[/code]This can really help with parsing in dates that have neglected to add their timezone to the data.
Timey Manipulation
Another common operation is to manipulate dates. Here are some common operations.
These can be found with more detail here.
[code language=”javascript”]let now = moment(); // Now
let nowInTwoHours = now.clone().add(2, ‘hours’); // Now in two hours
let chaining = now.clone().add(2, ‘hours’).add(3, ‘days’); // Now in 2 hours and 3 days.
let startOfDay = now.clone().startOf(‘day’) // set this date to 12:00am today
let startOfMonth = moment().startOf(‘month’); // set to first of this month 12:00am
let endOfYear = moment().endOf(‘year’); // 12-31 23:59:59.999 this year
[/code]
Displaying Dates
As you expect Moment can format dates into a string so that they are easy to read. Doc’s for that can be found here.
[code language=”javascript”]moment().format("dddd, MMMM Do YYYY, h:mm:ss a Z"); // "Sunday, February 14th 2010, 3:25:50 pm +13:00"
[/code]
Comparing Dates
Comparing dates is a very useful thing that Moment.js can help us with.
[code language=”javascript”]moment(‘2016-12-02’).isBefore(‘2017-12-02’); // true
moment(‘2010-10-20’).isBefore(‘2010-12-31’, ‘year’); // false
moment(‘2010-10-20’).isBefore(‘2011-01-01’, ‘year’); // true
moment([2011, 2, 12]).isDST(); // false, March 12 2011 is not DST
moment([2011, 2, 14]).isDST(); // true, March 14 2011 is DST
[/code]
Other things
Moment lots and lots of fun date/time related functions, and the ones you want will depend on the application you are developing. Best thing to do is browse through the docs and see if Moment can help.
Here are a few other fun functions
[code language=”javascript”]moment("2012-02", "YYYY-MM").daysInMonth() // 29
moment("2012-01", "YYYY-MM").daysInMonth() // 31
moment([2013, 11, 20]).toNow(); // in 4 years
moment(1318874398806).unix(); // 1318874398
moment(1318874398806).valueOf(); // 1318874398806
[/code]Also a good thing to note is Moment has many plugins if the base library does not cover your niche. See more here.
A big thanks to all the Moment developers for creating a library that handles the headaches of dates/times for us.
Hopefully this quick fly through of Moment gave you a clue on where to start for dealing with dates/times
Until next time
Tim Gray
Coffee to Code
Image Source: geralt / Pixabay